- sympy()
심볼릭 연산 / 인수분해, 미적분 등 기호 계산을 위한 오픈소스 파이썬 라이브러리
편미분 등 웬만한 수학 식 계산 가능
from sympy import *
import sympy
print(init_printing())
print(Rational(2,3))
print(pi) #심볼로서 존재
print(N(pi)) #넘버로 출력
print(pi.evalf())
#output
None
2/3
pi
3.14159265358979
3.14159265358979
from sympy import *
import sympy
x,y=sympy.symbols('x y')
f1=x+y
f2=x**3+3*x**2*y+3*x*y**2+y**3
print((f1**2).expand())
#expand() 없애면 (x + y)**2로 뜨지만, expand() 붙이면 전개식이 나옴
print((f2).factor())
#output
x**2 + 2*x*y + y**2
(x + y)**3
from sympy import *
import sympy
a,b,c=symbols('a,b,c')
f=Eq(a*x**2+b*x+c,0)
print(f)
print(solve(f, x)) #공식의 해를 나타냄.
print(solve(Eq(x**2,-4),x))
print("----------------------------")
print(diff(sin(x),x))
print(diff(x**3, x,x))
print(diff(x**3, x,3))
print(diff(y*x**3+x*y**2, x, y))
print("----------------------------")
import sympy
x, y = sympy.symbols("x y")
f = sympy.sqrt(x) + y*x**2
print(f)
print("f의 미분={}".format(sympy.diff(f, x)))
integrate(1/x,x)
integrate(6*x**2,(x,0,2))
integrate(exp(-x**2),(x,0,oo))
integrate(exp(-x**2-y**2),(x,-oo,oo),(y,-oo,oo))
import math
x, y = sympy.symbols("x y")
f = sympy.sin(x)
print("f의 정적분={}".format(sympy.integrate(f, (x,0,math.pi))))
from sympy import *
x= sympy.symbols("x")
f = (x-2)*x*(x+2)
a=solve(f)
print(a)
#output
Eq(a*x**2 + b*x + c, 0)
[(-b - sqrt(-4*a*c + b**2))/(2*a), (-b + sqrt(-4*a*c + b**2))/(2*a)]
[-2*I, 2*I]
----------------------------
cos(x)
6*x
6
3*x**2 + 2*y
----------------------------
sqrt(x) + x**2*y
f의 미분=2*x*y + 1/(2*sqrt(x))
f의 정적분=2.00000000000000
[-2, 0, 2]
A = Matrix( [[1,2,3],[4,5,6],[7,8,9]] )
print(A)
print(eye(3))
print(A*eye(3))
a11, a12, a21, a22 = symbols('a11, a12, a21, a22')
A = Matrix( [[a11, a12],[a21, a22]] )
print(A)
print(A.det())
print(A.inv())
from sympy import *
init_printing()
x, y = symbols("x y")
print(solve([2*x-y-5, x-y-2], [x,y]))
from sympy import *
A=Matrix([[2,-1], [1,-1]])
b=Matrix([5,2])
x=A.inv()*b
print(x)
----------------------------------------------------------
#output
Matrix([[1, 2, 3], [4, 5, 6], [7, 8, 9]])
Matrix([[1, 0, 0], [0, 1, 0], [0, 0, 1]])
Matrix([[1, 2, 3], [4, 5, 6], [7, 8, 9]])
Matrix([[a11, a12], [a21, a22]])
a11*a22 - a12*a21
Matrix([[a22/(a11*a22 - a12*a21), -a12/(a11*a22 - a12*a21)], [-a21/(a11*a22 - a12*a21), a11/(a11*a22 - a12*a21)]])
{x: 3, y: 1}
Matrix([[3], [1]])
- scipy()
수치 값 계산해서 알려줌
sp.constants.c: 빛의속도??뭐라는거임
import scipy as sp
import scipy.constants
print(sp.constants.c)
import matplotlib.pyplot as plt
from scipy.special import expit, logit
import numpy as np
x = np.linspace(-10, 10, 1000)
y = expit(x)
plt.plot(x, y)
plt.title("logistic sigmoid function")
plt.grid()
plt.xlabel('x')
plt.show()
import scipy.integrate
func= lambda x: x**2
integ_val = scipy.integrate.quad(func, 0, 100)
print (integ_val)
#output
299792458.0

from scipy import linalg
array1 = np.array([[3, 4],[5, 6]])
linalg.det(array1) #선형함수
iarray = linalg.inv(array1)
print(iarray)
#output
(333333.3333333333, 3.7007434154171883e-09)
[[-3. 2. ]
[ 2.5 -1.5]]
cubic,,은 음 점과 점 사이의 일은 모르나 예측해서 모양 보여줌.. 뭔 개소리지
input_time = np.linspace(0, 1, 10)
noise = (np.random.random(10)*2 - 1) * 1e-1
output = np.cos(2 * np.pi * input_time) + noise
from scipy.interpolate import interp1d
linear_interp = interp1d(input_time, output)
interp_time = np.linspace(0, 1, 200)
linear_output = linear_interp(interp_time)
cubic_interp = interp1d(input_time, output, kind='cubic')
cubic_output = cubic_interp(interp_time)
import matplotlib.pyplot as plt
plt.grid()
plt.plot(input_time,output,'o',interp_time,linear_output,'-',
interp_time,cubic_output,'--')
plt.legend(['data', 'linear', 'cubic'])
plt.show()
from scipy import stats
import matplotlib.pyplot as plt
x=[1,2,3,4,5,6,7,8,9]
y=[2,4,5,6,5,8,9,7,8]
weight, bias, r_value, p_value, std_err=stats.linregress(x,y)
def myfunc(x):
return weight*x+bias
mymodel=list(map(myfunc,x))
print("weght=",round(weight,3),"bias=",round(bias,3))
plt.grid()
plt.xlabel('x')
plt.plot(x,y,'o')
plt.plot(x,mymodel)
plt.show()
#output
weght= 0.717 bias= 2.417


from scipy.fftpack import fft
N = 500
T = 1.0 / 500.0
x = np.linspace(0.0, N*T, N)
y = np.sin(100.0 * 2.0*np.pi*x) + np.sin(200.0 * 2.0*np.pi*x)
yf = fft(y)
xf = np.linspace(0.0, 1.0/(2.0*T), N//2)
import matplotlib.pyplot as plt
plt.plot(xf, 2.0/N * np.abs(yf[0:N//2]))
plt.show()
print("-------------------------------")
# 1st order low-pass filter: H(s) = 10000 / (s + 1000)
from scipy import signal
import matplotlib.pyplot as plt
s1 = signal.lti([10], [0.001, 1])
w, mag, phase = signal.bode(s1)
plt.subplot(2,1,1)
plt.semilogx(w, mag) # Bode magnitude plot
plt.xlabel('frequency [rad/s]')
plt.ylabel('Magnitude[dB]')
plt.grid()
plt.title('Bode plot')
plt.subplot(2,1,2)
plt.semilogx(w, phase) # Bode phase plot
plt.xlabel('frequency[rad/s]')
plt.ylabel('Phase [deg]')
plt.grid()
plt.show()


~~~~기말최종공지~~~~
야호 다음주면 종강이당앙아
1. 1-14주차
2. 코드 5-6
3. 나머지는 중간이랑 동일
4. 종속. 상속문제. 채점 빡빡해질 예정.
5. 오픈북
6. 경로설정 파일만 넣어도 돌아가게끔??? / 경로 들어가는 순간 감점
파이썬 파일 이름만 넣어도(받게) 돌아가도록.
상대경로 절대불가. 절대경로만 ㄱㄱ
어라 이거 어케하라는 거?
7. 두시간 반
8. 출력하라 == print
9. Plot show 없이 저장만 요구하는 경우도 있으니 잘 확인. 그림출력-plot show, 값 출력-print
10. csv파일 이용 예정
~~~~교수의 한마디~~~~
기계
심층
강화
추천시스템
자연어 처리
빅데이터분석
자율이동체
지능형게임
생성형 ai
여기서 파이썬 쓴답니다
캠퍼스 특허 유니버사이드? 이런거 하신갑니당
차량통신 가상환경 로봇연동, 모빌리티 특허 문의 메일 ㄱㄴ
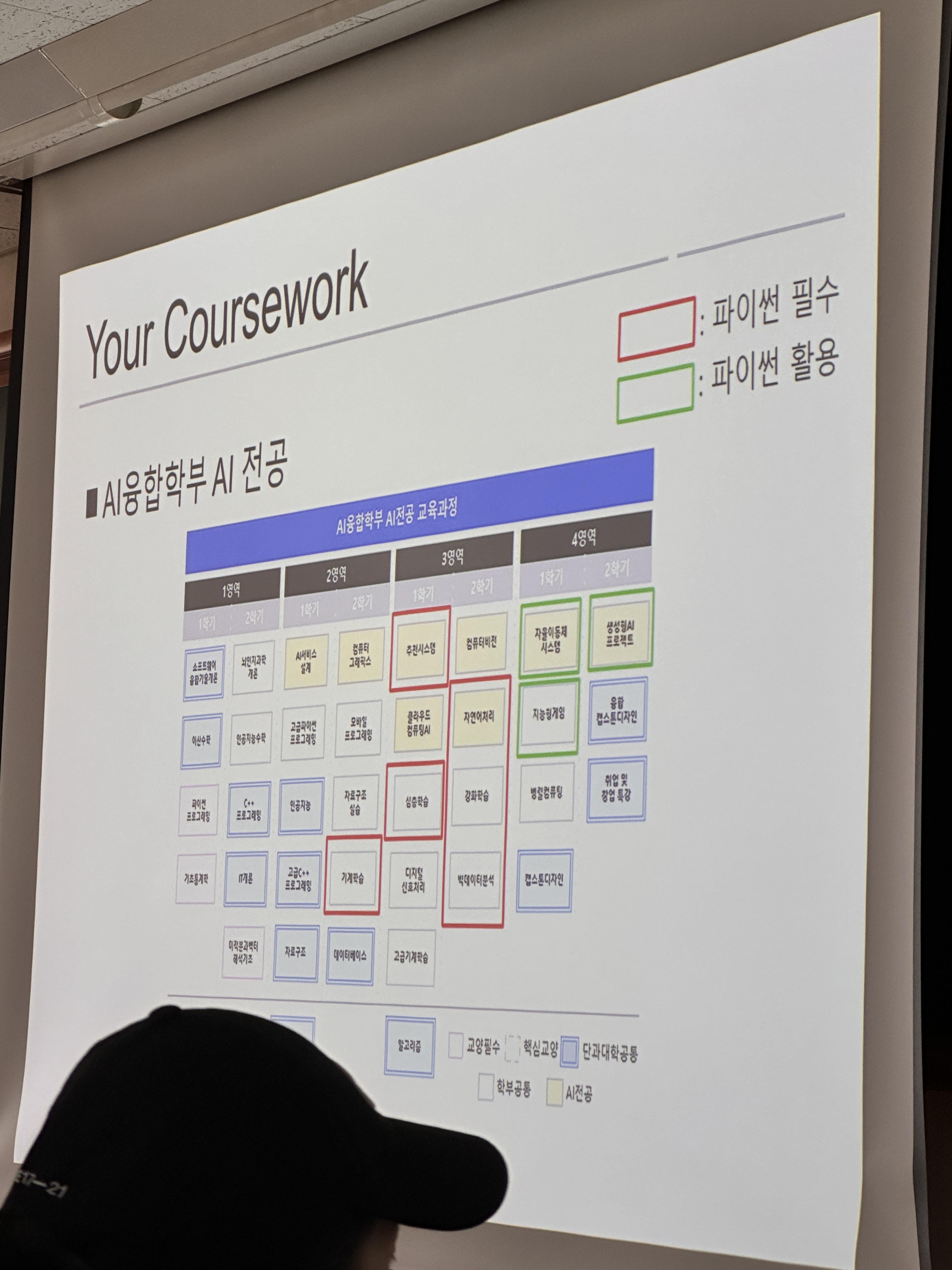

'Pworkspace' 카테고리의 다른 글
week10 - numpy(쉅안온날) (0) | 2024.05.28 |
---|---|
week13 - pandas(2), 과제5, 기말고사 공지(?) (0) | 2024.05.28 |
week12 - pandas (11주차에 조금 당겨서 배움) (0) | 2024.05.17 |
week11 - numpy(2)(histogram+ 너구리) (0) | 2024.05.14 |
week9 - matplotlib(시험범위 귀띔) (0) | 2024.04.30 |